As was previously mentioned, in order to display a variable in C, a format specifier must be used inside the printf function. The data type describes what kind and how much data the variable will hold. Here, we’ll concentrate on the most fundamental ones:
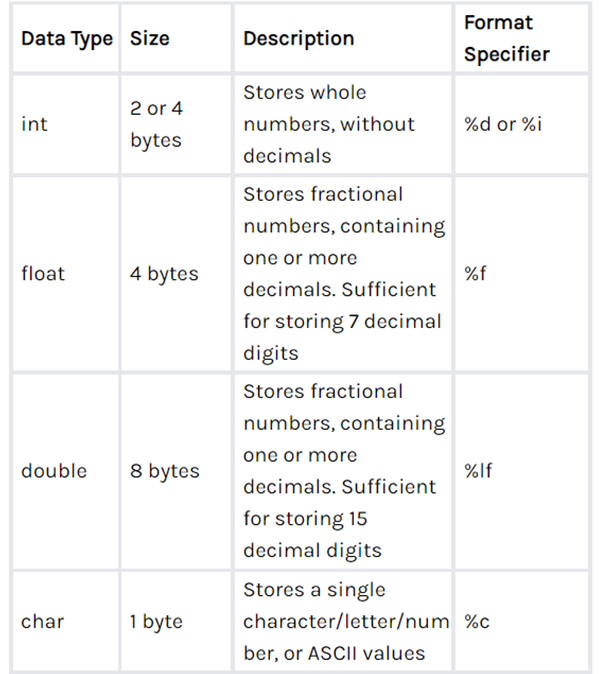
#include <stdio.h>
int main()
{
// Creating variables having different data types
int integer = 26;
float floating = 39.32;
char character = 'A';
// Printing variables with the help of their respective format specifiers
printf("%d\n", integer);
printf("%f\n", floating);
printf("%c\n", character);
}
Output: 26
39.320000
A
C constants
- Use the const keyword when using variables that you don’t want others or yourself to accidentally or purposefully change later in your application (this will declare the variable as “constant”, which means unchangeable and read-only).
- When a variable’s values are unlikely to change, such as any mathematical constant like PI, it should always be declared a constant.
- A constant variable must have a value before being declared.
This is an illustration of how to declare a constant.
#include <stdio.h>
int main()
{
const int MOD = 10000007;
}