Here is the program to sort the given integer in ascending order using insertion sort method. Please find the pictorial tutor of the insertion sorting.
Logic : Here, sorting takes place by inserting a particular element at the appropriate position, that’s why the name-Â insertion sorting. In the First iteration, second element A[1] is compared with the first element A[0]. In the second iteration third element is compared with first and second element. In general, in every iteration an element is compared with all the elements before it. While comparing if it is found that the element can be inserted at a suitable position, then space is created for it by shifting the other elements one position up and inserts the desired element at the suitable position. This procedure is repeated for all the elements in the list.
If we complement the if condition in this program, it will give out the sorted array in descending order. Sorting can also be done in other methods, like selection sorting and bubble sorting, which follows in the next pages.
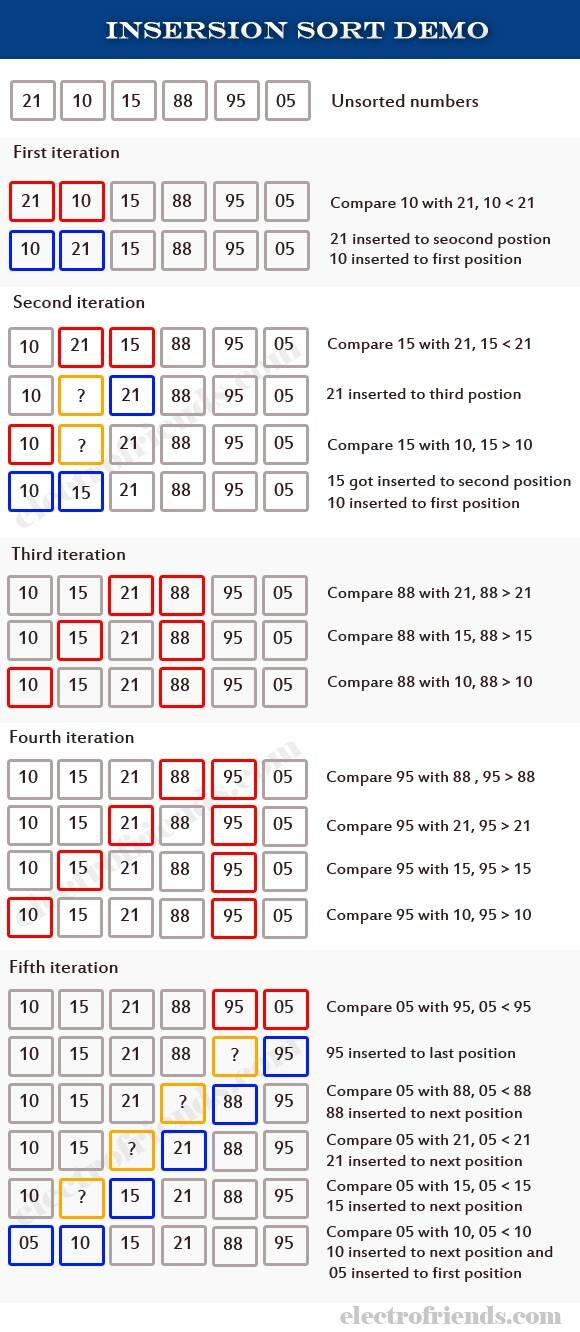
C program for Insertion Sort:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | #include void main() { int A[20], N, Temp, i, j; clrscr(); printf("\n\n\t ENTER THE NUMBER OF TERMS...: "); scanf("%d", &N); printf("\n\t ENTER THE ELEMENTS OF THE ARRAY...:"); for(i=0; i<N; i++) { scanf("\n\t\t%d", &A[i]); } for(i=1; i<N; i++) { Temp = A[i]; j = i-1; while(Temp<A[j] && j>=0) { A[j+1] = A[j]; j = j-1; } A[j+1] = Temp; } printf("\n\tTHE ASCENDING ORDER LIST IS...:\n"); for(i=0; i<N; i++) printf("\n\t\t\t%d", A[i]); getch(); } |
Hi,
Really good article for which neatly explains the sort. Good work guys. keep posting 🙂
Good explanation.. 🙂
This is not insertion sort, more like a selection sort.
In insertion sort we have to move the elements to make room, but i understand that u have implemented that using the comparison and swapping of each preceding element, but since we are building a sorted list with each insertion, we don’t have to compare any elements that occur before the smaller element(see in the third iteration 88 >21 so u can stop the comparison there and move to the next element).
realy, nice explanation….keep it up for other sorting methods…
nice one! it’s understandable when demonstrations are being presented… Good job!!! =)
c pseudocode is nt working
Fantastic article….great guys
nice easy explanation on insertion sort………i liked it………good job
enx in helping ne solve my problem in sorting it really work
keep posting for me ,somehow learn more!!! thanks:)
hello, thank you for posting easy sample of insertion sort, now i iderstant it well.
hey…excelent ….it ws really confusing me a lot…bt, this example gave me a great idea of how it xactly works…
thanks a lot ……. 🙂
Thanks may God bless you ur great work
Thanks a lot keep this good work going.
very easy method …………….
thanxs…….
its understood easily. thanks for helping out
fantastic explanation and help for exam
its actually thanks u and score t subject bcz f u guys…….!
u r briliant*********
very easy method…………
and simple language& soft commnication between checker and examinar
checker clear the concept……….bcz of me & u ………….!!!!
Your the great
hi , i’M Nadeem i checked your explaination and its really help full..
Thousand thanks to your kind effort to help guys like me+
Don’t you need to include conio.h?
Also, is Temp variable really necessary?
what is the work of this gotoxy(25,11+i); …..???
Hey ! u r awesome ……
u did a such a brilliant job.
i got full mark in my seminor..
thanks a lot
its really nice… compact but precise!
thanks guys,its really easy to learn,keep that
awesome explanation
GOOD Stuff !!!!! Well Done!!!
Nice article, and explanations
good stuff!!!!!!!!!!!!!!!!!!!!!!
keep goinng
hey , any one explain complexity of selection sort
m really impressed by pictorial representation of Insertion Sort
nice program ….thnkyou …
Really very nice explanation.
your implementation has small, but pretty serious bug.
check line: while (Temp = 0)
before it’s verified that j IS positive, A[] array can be indexed with negative offset.
i suggest to test programs with valgrind, it warns about memory overruns and underruns.
whoops, seems some symbols are ignored as possible HTML tags.
just check the while line then, and think about it
i like most uuurrrrrr site <<<>>>>>>>>>>>
Thnx thats a great explanation 😀
Superb explanation.Thank you,
But i have a question please any1 help me,
if i understand logic of programs such like this then should i memorise it
because it is hard to remember these logic and take alot of time if needed
after few weeks without practise!
hey friends,
i need u r help to improve in c .
well pls tel the how can i be best in this language
waiting 4 a nice help line
good article 🙂
perfect…… :putnam:
easily the best decsription of Insertion Sort on the web
keep up the good work
best of luck 🙂
IM LOOKING FOR NAMES TO BE SORTED NOT NUMBERS>>>>>
PLSSSS!!!!!!!!!!!!!!
it is very good explanation regarding insertion sort.
it is not confusing code
what do i need to change to make it in descending order?
You just have to change the loop as for(i=(n-1);i>=0;i–)
printf(“%d”,A[i]);
in the last loop.
awesome…
helps me a lot!!!!
its one of the good explain to know the basic of the insertion sort coding its help me to know about c
what code should be made to count the number of executions? please help..
it is not insertion sort rather it is SELECTION SORT