Here is the program to sort the given integer in ascending order using insertion sort method. Please find the pictorial tutor of the insertion sorting.
Logic : Here, sorting takes place by inserting a particular element at the appropriate position, that’s why the name-Â insertion sorting. In the First iteration, second element A[1] is compared with the first element A[0]. In the second iteration third element is compared with first and second element. In general, in every iteration an element is compared with all the elements before it. While comparing if it is found that the element can be inserted at a suitable position, then space is created for it by shifting the other elements one position up and inserts the desired element at the suitable position. This procedure is repeated for all the elements in the list.
If we complement the if condition in this program, it will give out the sorted array in descending order. Sorting can also be done in other methods, like selection sorting and bubble sorting, which follows in the next pages.
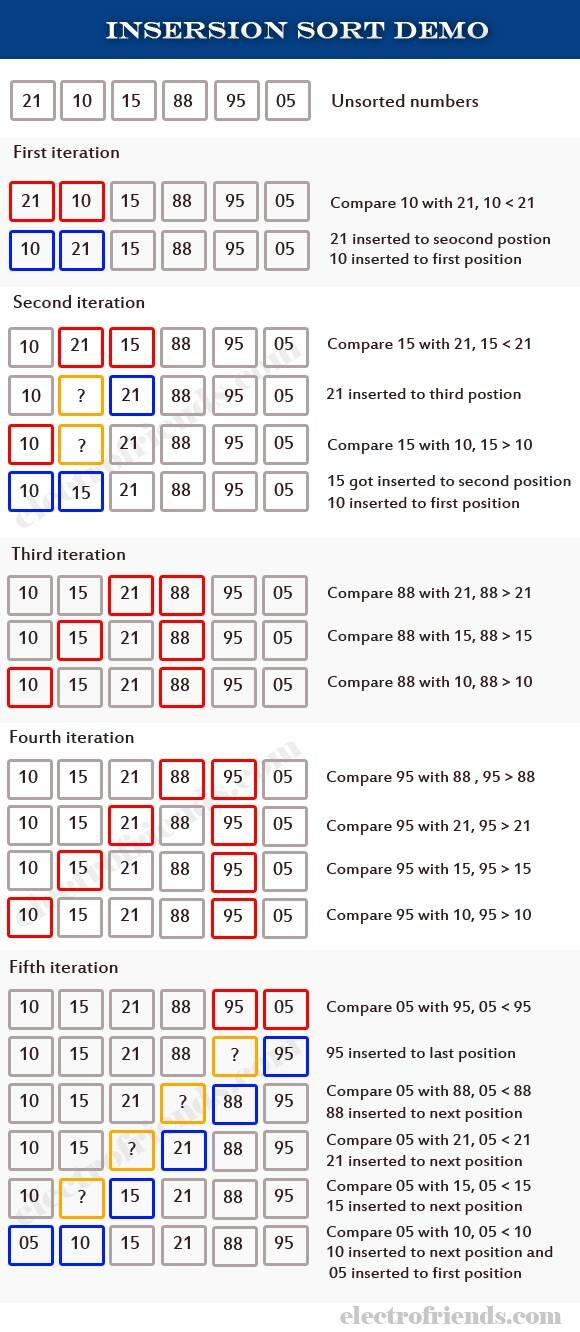
C program for Insertion Sort:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | #include void main() { int A[20], N, Temp, i, j; clrscr(); printf("\n\n\t ENTER THE NUMBER OF TERMS...: "); scanf("%d", &N); printf("\n\t ENTER THE ELEMENTS OF THE ARRAY...:"); for(i=0; i<N; i++) { scanf("\n\t\t%d", &A[i]); } for(i=1; i<N; i++) { Temp = A[i]; j = i-1; while(Temp<A[j] && j>=0) { A[j+1] = A[j]; j = j-1; } A[j+1] = Temp; } printf("\n\tTHE ASCENDING ORDER LIST IS...:\n"); for(i=0; i<N; i++) printf("\n\t\t\t%d", A[i]); getch(); } |
Great Explanation. I had read insertion sort so many times, but could not understand it, your example was superb. Thanks a lot!
It’s small,simple. Even though logic is big you write the prog in small.
very nice explanation….easy to understand
Thanks for your great explanation with an apt example.
And here i am having a question what is the use of gotoxy
and how is this working
thanks for great explanation………
i actually didnt understand the line gotoxy(25,11+i);
is it a built in function?
thanku for the xplanation… it was of gr8 help..
#include
#include
using namespace std;
const int SIZE = 20;
int binarySearch(string [], int, int);
void selectionSort (string[], int);
int main()
{
string nameSearch;
string name[SIZE] =
{“Collins, Bill”, “Smith, Bart”, “Michalski, Joe”, “Griffen, Jim”,
“Sanchez, Manny”, “Rubin, Sarah”, “Taylor, Tyrone”, “Johnson, Jill”,
“Allison, Jeff”, “Moreno, Juan”, “Wolfe, Bill”, “Whitman, Jean”,
“Moretti, Bella”, “Wu, Hong”, “Patel, Renee”, “Harrison, Rose”,
“Smith, Cathy”, “Conroy, Pat”, “Kelly, Sean”, “Holland, Beth”};
cout <> nameSearch;
return 0;
}
int binarySearch(string array[], int size, int value)
{
int first = 0,
last = size – 1,
middle,
position = -1;
bool found = false;
while (!found && first value)
last = middle – 1;
else
first = middle + 1;
}
return position;
}
void selectionSort(string array[], int size)
{
int startScan, minIndex, minValue;
for(startScan = 0; startScan < (size – 1); startScan++)
{
minIndex = startScan;
minValue = array[startScan];
for (int index = startScan + 1; index < size; index++)
{
if (array[index] < minValue)
{
counter++;
minValue = array[index];
minIndex = index;
}
}
array[minIndex] = array[startScan];
array[startScan] = minValue;
}
return counter;
}
why do you need to add “&& j>=0” on the 18th row?
thank u, it’s simple nd more understanding, especially d apt work, dat
is useful to understand more.
Thnx man gr8 work … it’s a fine example..mindblowing
#include
#include
void insertsort(int ar[],int n)
{
int i,j,t;
for(i=1;it)
{
ar[j+1]=ar[j];
j–;
}
ar[j+1]=t;
}
}
void main()
{
int ar[10],i;
clrscr();
ar[0]=-32768;
for(i=1;i<=9;i++)
{
scanf("%d",&ar[i]);
}
insertsort(ar,10);
printf("\nSorted data\n");
for(i=1;i<=9;i++)
{
printf("%d ",ar[i]);
}
getch();
}
it’s Nise!
please include more metheds
it’s Nise!
please include more metheds
thankyou
Thanks Sir..
I cleared my concept.
this pgm not working in kdevelop i understand logic in up page shown but not in pgm
thank you sir.
realy,it’s very good explanation….
Thanks
thanks for the explanation … but i have a question ? what are the
codes for each pass…
thank u explanation is very neice
it helps me alot…tnx
void swap(int *src, int *dest)
{
int temp;
temp = *src;
*src = *dest;
*dest = temp;
}
main ()
{
int a[] = {4,8,7,3,2,9,0,1,6,5};
unsigned int n = sizeof(a)/sizeof(a[0]);
int i=0, j=0;
for(i=1; i=0; j–) {
if(a[j] > a[j+1]) {
swap(&a[j+1], &a[j]);
}
}
}
for(i=0; i<n; i++)
printf("Sorted list : %d ", a[i]);
return 0;
}
thankx sir ye program to hmara run hi nahi ho raha tha but now i done it
Its very helpful for me,now every confusion has been finished…………..so thank you so so …………..much.
Its very helpful for me,now every confusion has been cleared…………..so thank you so so …………..much.
just WOWWW…
Your diagram and code doestnt match!
Program is good, but not diagram.
#include
void main()
{
int A[20], N, Temp, i, j;
clrscr();
printf(“\n\n\t ENTER THE NUMBER OF TERMS…: “);
scanf(“%d”, &N);
printf(“\n\t ENTER THE ELEMENTS OF THE ARRAY…:”);
for(i=0; i<N; i++)
{
gotoxy(25,11+i);
scanf("\n\t\t%d", &A[i]);
}
for(i=1; i<N; i++)
{
Temp = A[i];
j = i-1;
while(Temp=0)
{
A[j+1] = A[j];
j = j-1;
}
A[j+1] = Temp;
}
printf(“\n\tTHE ASCENDING ORDER LIST IS…:\n”);
for(i=0; i<N; i++)
printf("\n\t\t\t%d", A[i]);
getch();
}
thank u . . .Its is very useful . . .Keep posting more codes . .
i want print like this
.1
A B
2 3 4
C D E F . . . .like this any one help me write the coding for that
Sir. can you do a dutch flag problem using a insertion sort?
thanks
kya hai.. kuch output nai ara hai
simulation of sorting (all sorting)
simulation of sorting(all sorting) progam in c language
i need a unique & short program using insertion sort ?? can you help me ?
hii..i want to know the meaning of why we use j=j-1 after assigning temp to a[i] in insertion sort..please explain it as soon as possible my mail id is gchetna30@gmail.com.
main()
#define size 10
{
int arr[size],i,j,item;
printf(“\n enter the element in array”);
for(i=0;i<size;i++)
scanf("%d",&arr[i]);
for(j=0;j<size;j++)
{ item=arr[i];
for(i=j-1;item=0;i–)
{ arr[i+1]=arr[i];
arr[i]=item;
}}
thnxx.
i understood insertion stood.
For balchhal programs contact AOT guys….West bengal…chodao bara
Rohon dar gude batha…tai boli dudu khao..
sir,i want to know what is use of gotoxy()?
multy way tree
#include
#include
void main()
{
int a[20],k,n,i=0,j=0,t=0;
clrscr();
printf(“no of of elemts”);
scanf(“%d”,&n);
printf(“enter %d values”,n);
for(i=0;i<n;i++)
scanf("%d",&a[i]);
for(i=1;i=1;j–)
{
printf(“i=%d j=%d %d %d”,i,j,a[j],a[j-1]);
if(a[j]>a[j-1])
break;
else
{
t=a[j];
a[j]=a[j-1];
a[j-1]=t;
}
} printf(“\n”);
} printf(“\n”);
for(i=0;i<n;i++)
printf(" %d ",a[i]);
getch();
}
THE ABOVE CODE REPRESENTS WHICH SORTING TECHNIQUE????
DOES IT REPRESENT INSERTION SORT????
m confused in insertion sort …..when comparison is done and 1st pass completes then how elements compare with all the previous elements there is no loop ……pls explain…..
Well explained. I assume there is one error in the code during runtime. Changed below code.
while( j>=0 && Temp<A[j] )
{
A[j+1] = A[j];
j = j-1;
}
thanks
#include
using namespace std;
int main()
{ int k,A[6];
for(int i=0;i>A[i];
}
for(int i=1;i=0 && A[j]>A[i])
{
A[j+1]=A[j];
j–;
}
A[j+1]=value;
}
cout<<"sorted list"<<endl;
for( k=0;k<6;k++)
{
cout<<A[k]<<"\t";
}
return 0;
}
/* wht give the wrong output?? i tryed to check it …plz help*/
Source code of simple merge sort implementation using array in ascending order in c programming language
#include
#define MAX 50
void mergeSort(int arr[],int low,int mid,int high);
void partition(int arr[],int low,int high);
int main(){
int merge[MAX],i,n;
printf(“Enter the total number of elements: “);
scanf(“%d”,&n);
printf(“Enter the elements which to be sort: “);
for(i=0;i<n;i++){
scanf("%d",&merge[i]);
}
partition(merge,0,n-1);
printf("After merge sorting elements are: ");
for(i=0;i<n;i++){
printf("%d ",merge[i]);
}
return 0;
}
void partition(int arr[],int low,int high){
int mid;
if(low<high){
mid=(low+high)/2;
partition(arr,low,mid);
partition(arr,mid+1,high);
mergeSort(arr,low,mid,high);
}
}
void mergeSort(int arr[],int low,int mid,int high){
int i,m,k,l,temp[MAX];
l=low;
i=low;
m=mid+1;
while((l<=mid)&&(m<=high)){
if(arr[l]mid){
for(k=m;k<=high;k++){
temp[i]=arr[k];
i++;
}
}
else{
for(k=l;k<=mid;k++){
temp[i]=arr[k];
i++;
}
}
for(k=low;k<=high;k++){
arr[k]=temp[k];
}
}
Sample output:
Enter the total number of elements: 5
Enter the elements which to be sort: 2 5 0 9 1
After merge sorting elements are: 0 1 2 5 9