Here is the program to sort the given integer in ascending order using bubble sort method. Please find the pictorial tutor of the bubble sorting.
Logic : The entered integers are stored in the array A. Here, to sort the data in ascending order, any number is compared with the next numbers for orderliness. i.e. first element A[0] is compared with the second element A[1]. If forth is greater than the prior element then swapping them, else no change. Then second element is compared with third element, and procedure is continued. Hence, after the first iteration of the outer for loop, largest element is placed at the end of the array. In the second iteration, the comparisons are made till the last but one position and now second largest element is placed at the last but one position. The procedure is traced till the array length.
If we complement the if condition in this program, it will give out the sorted array in descending order. Sorting can also be done in other methods, like selection sorting and insertion sorting, which follows in the next pages.
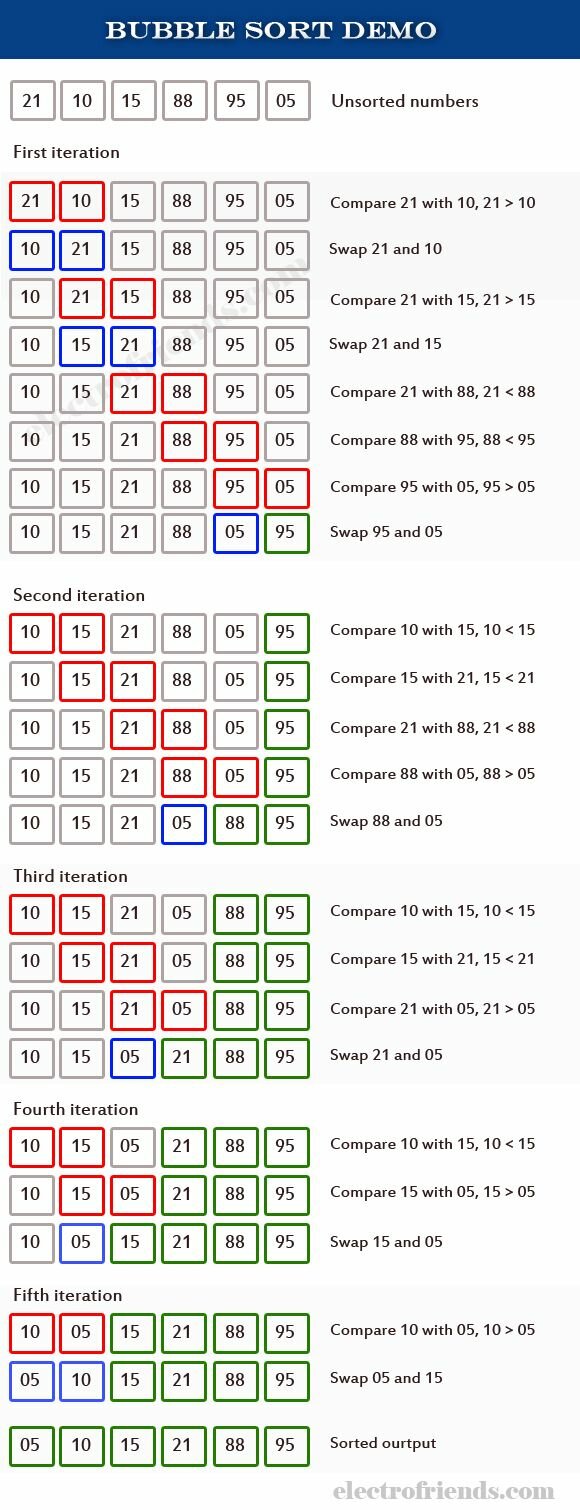
Here is the C program to sort the numbers using Bubble sort
#include void main() { int A[20], N, Temp, i, j; clrscr(); printf(“\n\n\t ENTER THE NUMBER OF TERMS…: “); scanf(“%d”,&N); printf(“\n\t ENTER THE ELEMENTS OF THE ARRAY…:”); for(i=0; i<N; i++) { scanf(“\n\t\t%d”, &A[i]); } for(i=0; i<N-1; i++) for(j=0; j<N-i;j++) if(A[j]>A[j+1]) { Temp = A[j]; A[j] = A[j+1]; A[j+1] = Temp; } printf(“\n\tTHE ASCENDING ORDER LIST IS…:\n”); for(i=0; i<N; i++) printf(“\n\t\t\t%d”,A[i]); getch(); } |
Program is nice…
but very typical to read….
try to use CODE TAG …
it s very nice .you have cleared all my doubts.its to the point yet very helpful.
ya…nice 2 understand….very helpful
clear….eay to understand
Thank you so much ..for the info really helpful
Btw how can i put the descending order along with the ascending???
output
Ascending order is: Descending order is
1 3
2 2
3 1
i tried duplicating it below before getch and changed < to greater than to i but still shows the same sort in ascending…Pls2x reply 😉
ohh i figured it out myself 😀 thanks anyway 😀
for the initial info 😀
#include
#include
void main()
{
int A[20],N,Temp,i,j;
clrscr();
printf(“\n\n\t Enter the number of terms:”);
scanf(“%d”,&N);
printf(“\n\t Enter the elements of the array”);
for(i=1;i<=N;i++)
{
gotoxy(25,5+i);
scanf("\n\t\t%d",&A[i]);
}
for(i=1;i<=N-1;i++)
for(j=1;jA[j+1])
{
Temp = A[j];
A[j] =A[j+1];
A[j+1] = Temp;
}
printf(“\n\t the Ascending order list is:\n”);
for(i=1; i to < */
/*———————————————————-*/
for(i=1;i<=N-1;i++)
for(j=1;j<=N-i;j++)
if (A[j]<A[j+1])
{
Temp = A[j];
A[j] =A[j+1];
A[j+1] = Temp;
}
printf("\n\t the Descending order list is:\n");
for(i=1;i<=N;i++)
printf("\n\t\t\t%d",A[i]);
getch();
}
Starting from the line:D i added the descending sort 😀 if anyone needs it there you have it 😀
Sorry if my English is bad …..
and by the way since its not shown what the includes are cause it may cause some errors
its include
include
Have a nice day evry1:D
ohh my its still not shown
maybe i just type the
insides of the prototype
stdio.h and conio.h………
ya it is gud
very helpful fo me to do it easily thankss………:):P 😀
This one is also a great work .. thanks
this information is very helpful for not only students even also teachers. simply its too helpful.
FOR ME its very helpful.
very nice ..
the information is very useful. THANKS FOR THIS
Hey Ranjith,
Thanks for the good work. Bubble sort algorithm should check whether swap occured atleast once if not the array is sorted and the algo should stop :D.
this is a good program within a small amount of code………………
thank you for your best companion
The second for loop should be N-1-i like below
for(j=0; j<(N-1)-i;j++)
thanks a lot,i like this.this is good program with good example but
The second for loop should be N-1-i like below
for(j=0; j<(N-1)-i;j++)
Please can you help me write this in BASIC programing language? And also give the algorithm
Bubble sort is really an appalling algorithm and should never, ever, be used in production code.
From Wikipedia:
“However, some researchers such as Owen Astrachan have gone to great lengths to disparage bubble sort and its continued popularity in computer science education, recommending that it no longer even be taught.”
Donald Knuth, in his famous book The Art of Computer Programming, concluded that “the bubble sort seems to have nothing to recommend it, except a catchy name and the fact that it leads to some interesting theoretical problems”
why u r using the temp???
int pass,i;
float A[],n;
for(pass=1;pass<n;pass++)
{
for(i=0;iA[i+1])
swap(&A[i],&A[i+1]);
}
why in loop we are taking N-1 and N-i in suome side it is taken N-2.IAM not able to distinguish between in all these thing specially confused between selection sort,insertion sort and bubble sort.when to use N-1 when N-2 ETC.
thanks for uploading program….it helps me in the exam….just copy paste dude….
thank u very much.
y u used 25,11=I
Gold is one of the traditional precious metals used for wedding jewelry.
So how can the happy couple reduce the unrivalled stress levels that come with such an predominant event in their lives.
As a wedding is a one-time event, the photographer must be prepared for the unexpected.
The equipment itself is not really particularly expensive these times,
so leasing can cost considerably more in the medium-to-long term.
You can know all the ins and outs of business and can run a restaurant without fail; but would you know the first thing about installing the kitchen grills.
Two: Increase in income – When you are able to increase your
traffic to the business, you will easily be able to also increase
your income.
Starting a small business with no money may sound impossible but there are ways to kick off a very profitable
business without ever spending a single dime of your own money.
You can know all the ins and outs of business and can run a restaurant without fail;
but would you know the first thing about installing the
kitchen grills. Clients do not look no matter whether you’ve a small or big budgeted communication service.
The equipment itself is not really particularly expensive these
times, so leasing can cost considerably more in the medium-to-long term.
When job offers do come and I’ll admit, some companies may fear hiring you because they
fear a former owner being an employee, negotiate the best salary you can along with the best benefits you can for
you and your family. What you give away doesn’t have to be costly, but it must be valuable.
The equipment itself is not really particularly expensive these times,
so leasing can cost considerably more in the medium-to-long term.
Without enough traffic, you will never have enough
customers and this means you won’t be making enough money.
For clients, you can target neighborhoods, communities
or even companies.
The following are the benefits that you have to be aware of
the most. You can know all the ins and outs of business and can run a
restaurant without fail; but would you know the first thing about installing the kitchen grills.
Two: Increase in income – When you are able to increase your traffic to the business, you will easily be able to also
increase your income.
If you’re educated (or seeking an education) you will probably find a ton of opportunity in a
small town. As with everything else, it is only the mindset of the entrepreneur that dictates the success
and failure of a company. For clients, you can target neighborhoods,
communities or even companies.
Or, perhaps you’re one of those students who desires a challenge, or even a
good addition to your résumé for Grad school.
You can know all the ins and outs of business and can run a restaurant without fail; but
would you know the first thing about installing the kitchen grills.
Typically, this works well if we are involved in a solid networking and we reciprocate, by referring clients to those
other businesses.
Yet, there are grants that are made for equipment and
training. You can know all the ins and outs of business and
can run a restaurant without fail; but would you know the first thing about installing the
kitchen grills. Clients do not look no matter whether you’ve a small or big
budgeted communication service.
Then send the postcard to all of the addresses in your area, and try to draw customers in.
Platforms: Android, Blackberry, i – Phone, i
– Pad, Windows Phone. For clients, you can target neighborhoods, communities or even companies.
Yet, there are grants that are made for equipment and training.
A lot of people have small business ideas that they often fail to put into
practice either because they lack the money to make an investment or because the fear to
lose everything. When travelling, for instance, it would be
possible to login to your accounting database and enter transactions on the road.
Or, perhaps you’re one of those students who desires a challenge, or even a good
addition to your résumé for Grad school. Further, amount of
loan must be need-based, subject to ceiling of Rs 25,000 per borrower for purchase of machinery or equipment etc,
and meeting working capital requirement of one operating cycle.
Two: Increase in income – When you are able to increase your traffic
to the business, you will easily be able to also increase your income.
thanks for this
but could you just tell me the difference between insertion and bubble sort.