The PHP mail() function is used to send emails from inside a script.
Syntax:
mail(to,subject,message,headers,parameters)
Here is an example of PHP feedback-form which you can directly publish on your website. The example below sends a message to a specified e-mail address. This PHP form includes Name, Email ID, Phone No, Subject, Message. Here JavaScript validation is used for Name and email id.
HTML form:
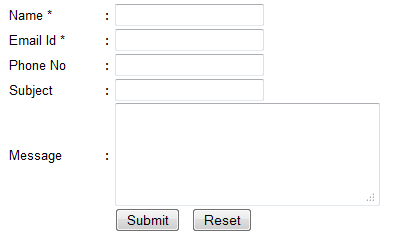
HTML form code:
This html code also includes JavaScript validation. Save this code as feedback.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Send enquiry - Electrofriends.com</title> <script language="javascript"> function checkField(f1, tbl1, tbl2) { if((f1.name.value=="") ) { document.getElementById(tbl1).style.display = ''; return false; } else if((f1.email.value=="") ) { document.getElementById(tbl2).style.display = ''; return false; } else return true; } </script> </head> <body> <form name="f1" method="post" action="sendemail.php"> <table width="522" border="0" align="center" cellpadding="0" cellspacing="0" style="font-family:Arial, Helvetica, sans-serif; font-size:10pt;"> <tr> <td width="16" height="25"> </td> <td width="96">Name *</td> <td width="10" height="25"><strong>:</strong></td> <td width="163" height="25"><input name="name" type="text" id="name" /></td> <td width="237" height="25"> <table width="233" border="0" cellspacing="0" cellpadding="0" id="tbl1" style="display:none; color:#FF0000"> <tr> <td>Enter the Name</td> </tr> </table> </td> </tr> <tr> <td height="25"> </td> <td height="25">Email Id *</td> <td height="25"><strong>:</strong></td> <td height="25"><input name="email" type="text" id="email" /></td> <td height="25"> <table width="233" border="0" cellspacing="0" cellpadding="0" id="tbl2" style="display:none; color:#FF0000"> <tr> <td>Enter the Email Id</td> </tr> </table> </td> </tr> <tr> <td height="25"> </td> <td height="25">Phone No</td> <td height="25"><strong>:</strong></td> <td height="25"><input name="phone" type="text" id="phone" /></td> <td height="25"> </td> </tr> <tr> <td height="25"> </td> <td height="25">Subject</td> <td height="25"><strong>:</strong></td> <td height="25"><input name="sub" type="text" id="sub" /></td> <td height="25"> </td> </tr> <tr> <td height="95"> </td> <td height="95">Message</td> <td height="95"><strong>:</strong></td> <td height="95" colspan="2"><textarea name="message" cols="30" rows="5" id="message"> </textarea></td> </tr> <tr> <td height="25" colspan="2"> </td> <td height="25"> </td> <td height="25" colspan="2"><input name="" type="submit" value="Submit" onclick="return checkField(f1,'tbl1','tbl2');" /> <input name="input4" type="reset" value="Reset" /></td> </tr> </table> </form> </body> </html> |
PHP code:
Save below code as sendemail.php and update your email id.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 | <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Send Mail | studentprojects.in</title> </head> <body> <?php $name = $_REQUEST["name"]; $email= $_REQUEST["email"]; $phone = $_REQUEST["phone"]; $sub= $_REQUEST["sub"]; $message = $_REQUEST["message"]; $todayis = date("l, F j, Y, g:i a") ; $subject = "Enquiry From Website " ; $to= "xyz@yourdomain.com"; $msg.= "<table width='500' border='0' align='center' cellpadding='0' cellspacing='0' style='font-family:Arial, Helvetica, sans-serif; font-size:10pt; border:1px solid #ccc;'> "; $msg.= "<tr>"; $msg.= "<td width='16' height='25'> </td>"; $msg.= "<td width='96'>Name</td>"; $msg.= "<td width='10' height='25'><strong>:</strong></td>"; $msg.= "<td height='25'>$name</td>"; $msg.= "</tr>"; $msg.= "<tr>"; $msg.= "<td height='25' bgcolor='#F5F5F5'> </td>"; $msg.= "<td height='25' bgcolor='#F5F5F5'>Email Id </td>"; $msg.= "<td height='25' bgcolor='#F5F5F5'><strong>:</strong></td>"; $msg.= "<td height='25' bgcolor='#F5F5F5'>$email</td>"; $msg.= "</tr>"; $msg.= "<tr>"; $msg.= "<td height='25'> </td>"; $msg.= "<td height='25'>Phone No</td>"; $msg.= "<td height='25'><strong>:</strong></td>"; $msg.= "<td height='25'>$phone</td>"; $msg.= "</tr>"; $msg.= "<tr>"; $msg.= "<td height='25' bgcolor='#F5F5F5'> </td>"; $msg.= "<td height='25' bgcolor='#F5F5F5'>Subject</td>"; $msg.= "<td height='25' bgcolor='#F5F5F5'><strong>:</strong></td>"; $msg.= "<td height='25' bgcolor='#F5F5F5'>$sub</td>"; $msg.= "</tr>"; $msg.= "<tr>"; $msg.= "<td height='95'> </td>"; $msg.= "<td height='95'>Message</td>"; $msg.= "<td height='95'><strong>:</strong></td>"; $msg.= "<td height='95'>$message</td>"; $msg.= "</tr>"; $msg.= "</table>"; $headers = "From: $name < $email >."; $headers .= "\r\nContent-Type: text/html; charset=ISO-8859-1\r\n"; mail($to, $subject, $msg, $headers) ; echo "<center><div align='center'>"; echo "<h1 style='font-family:arial, verdana; font-size:15pt;'>Your enquiry has been sent successfully!</h1>"; echo " <br /> "; ?> </body> </html> |
hay its working………….
and its very simple to use………..
thank you…………………………….
good code thank you…………..
nice article..
it does not work code works but mail not sent at desired mail address….
This code is not working..I am the php beginner..i must want the enquiry form code ..
Thanks verrrry much for d code, Working Perfectly.
Code dosen’t work,please help me i need it..please help me it showing the following error after submit..
Warning: date() [function.date]: It is not safe to rely on the system’s timezone settings. You are *required* to use the date.timezone setting or the date_default_timezone_set() function. In case you used any of those methods and you are still getting this warning, you most likely misspelled the timezone identifier. We selected ‘Asia/Kolkata’ for ‘5.5/no DST’ instead in C:\wamp\www\sendemail.php on line 18
Warning: mail() [function.mail]: Failed to connect to mailserver at “localhost” port 25, verify your “SMTP” and “smtp_port” setting in php.ini or use ini_set() in C:\wamp\www\sendemail.php on line 61
Your enquiry has been sent successfully!
Warning: date() [function.date]: It is not safe to rely on the system’s timezone settings. You are *required* to use the date.timezone setting or the date_default_timezone_set() function. In case you used any of those methods and you are still getting this warning, you most likely misspelled the timezone identifier. We selected ‘Asia/Kolkata’ for ‘5.5/no DST’ instead in C:\wamp\www\sendemail.php on line 18
Warning: mail() [function.mail]: Failed to connect to mailserver at “localhost” port 25, verify your “SMTP” and “smtp_port” setting in php.ini or use ini_set() in C:\wamp\www\sendemail.php on line 61
Your enquiry has been sent successfully!